mirror of
https://github.com/writeas/writefreely-swiftui-multiplatform.git
synced 2024-11-15 01:11:02 +00:00
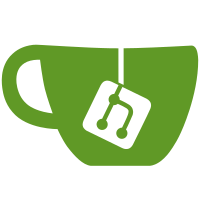
* Add error handling to Mac app * Log fatal crashes and present alert on next launch * Update crash alert copy and navigate to help forum * Refactor logging into reuseable methods * Refactor class to use protocol * Add environment object to settings window * Improve deactivation of app when miniaturizing * Chagne dispatch type when creating new post * Bump version and build number * Remove unnecessary TODO comment * Update change log * Log fatal crashes and present alert on next launch * Update crash alert copy and navigate to help forum * Refactor logging into reuseable methods * Add environment object to settings window * Improve deactivation of app when miniaturizing * Chagne dispatch type when creating new post * Improve default window size (#220) * Clean up unnecessary import * Set idealWidth property on sidebars * Unset selected post on collection change (#218) * Unset selected post when changing collection * Update change log * Bump build number and update change log
70 lines
2.9 KiB
Swift
70 lines
2.9 KiB
Swift
import SwiftUI
|
|
|
|
struct CollectionListView: View {
|
|
@EnvironmentObject var model: WriteFreelyModel
|
|
@EnvironmentObject var errorHandling: ErrorHandling
|
|
@FetchRequest(sortDescriptors: []) var collections: FetchedResults<WFACollection>
|
|
@State var selectedCollection: WFACollection?
|
|
|
|
var body: some View {
|
|
List(selection: $selectedCollection) {
|
|
if model.account.isLoggedIn {
|
|
NavigationLink("All Posts", destination: PostListView(selectedCollection: nil, showAllPosts: true))
|
|
NavigationLink(
|
|
model.account.server == "https://write.as" ? "Anonymous" : "Drafts",
|
|
destination: PostListView(selectedCollection: nil, showAllPosts: false)
|
|
)
|
|
Section(header: Text("Your Blogs")) {
|
|
ForEach(collections, id: \.self) { collection in
|
|
NavigationLink(destination: PostListView(selectedCollection: collection, showAllPosts: false),
|
|
tag: collection,
|
|
selection: $selectedCollection,
|
|
label: { Text("\(collection.title)") })
|
|
}
|
|
}
|
|
} else {
|
|
NavigationLink(destination: PostListView(selectedCollection: nil, showAllPosts: false)) {
|
|
Text("Drafts")
|
|
}
|
|
}
|
|
}
|
|
.navigationTitle(
|
|
model.account.isLoggedIn ? "\(URL(string: model.account.server)?.host ?? "WriteFreely")" : "WriteFreely"
|
|
)
|
|
.listStyle(SidebarListStyle())
|
|
.onChange(of: model.selectedCollection) { collection in
|
|
model.selectedPost = nil
|
|
if collection != model.editor.fetchSelectedCollectionFromAppStorage() {
|
|
self.model.editor.selectedCollectionURL = collection?.objectID.uriRepresentation()
|
|
}
|
|
}
|
|
.onChange(of: model.showAllPosts) { value in
|
|
model.selectedPost = nil
|
|
if value != model.editor.showAllPostsFlag {
|
|
self.model.editor.showAllPostsFlag = model.showAllPosts
|
|
}
|
|
}
|
|
.onChange(of: model.hasError) { value in
|
|
if value {
|
|
if let error = model.currentError {
|
|
self.errorHandling.handle(error: error)
|
|
} else {
|
|
self.errorHandling.handle(error: AppError.genericError())
|
|
}
|
|
model.hasError = false
|
|
}
|
|
}
|
|
}
|
|
}
|
|
|
|
struct CollectionListView_LoggedOutPreviews: PreviewProvider {
|
|
static var previews: some View {
|
|
let context = LocalStorageManager.standard.container.viewContext
|
|
let model = WriteFreelyModel()
|
|
|
|
return CollectionListView()
|
|
.environment(\.managedObjectContext, context)
|
|
.environmentObject(model)
|
|
}
|
|
}
|