mirror of
https://github.com/writeas/writefreely-swiftui-multiplatform.git
synced 2024-11-15 01:11:02 +00:00
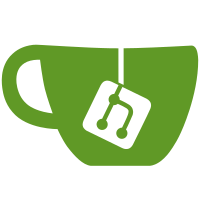
* Update Sparkle to latest version * Bump minimum macOS target For launch, I propose we support the current version of macOS (14.x) and one version earlier (13.x). * Add WFNavigation wrapper to use NavigationSplitView in macOS * Replace NavigationView with WFNavigation in ContentView * Fix deprecation warnings on locale * Update docs for updating the Mac app * Fix for being sent back to post list on app reactivate * Bump build version * Remove debugging statements * Bump Sparkle version to address security fix
113 lines
4.2 KiB
Swift
113 lines
4.2 KiB
Swift
import SwiftUI
|
|
|
|
struct ContentView: View {
|
|
@EnvironmentObject var model: WriteFreelyModel
|
|
@EnvironmentObject var errorHandling: ErrorHandling
|
|
|
|
var body: some View {
|
|
#if os(macOS)
|
|
WFNavigation(
|
|
collectionList: {
|
|
CollectionListView()
|
|
.withErrorHandling()
|
|
.toolbar {
|
|
if #available(macOS 13, *) {
|
|
EmptyView()
|
|
} else {
|
|
Button(
|
|
action: {
|
|
NSApp.keyWindow?.contentViewController?.tryToPerform(
|
|
#selector(NSSplitViewController.toggleSidebar(_:)), with: nil
|
|
)
|
|
},
|
|
label: { Image(systemName: "sidebar.left") }
|
|
)
|
|
.help("Toggle the sidebar's visibility.")
|
|
}
|
|
Spacer()
|
|
Button(action: {
|
|
withAnimation {
|
|
// Un-set the currently selected post
|
|
self.model.selectedPost = nil
|
|
}
|
|
// Create the new-post managed object
|
|
let managedPost = model.editor.generateNewLocalPost(withFont: model.preferences.font)
|
|
withAnimation {
|
|
DispatchQueue.main.async {
|
|
// Load the new post in the editor
|
|
self.model.selectedPost = managedPost
|
|
}
|
|
}
|
|
}, label: { Image(systemName: "square.and.pencil") })
|
|
.help("Create a new local draft.")
|
|
}
|
|
.frame(width: 200)
|
|
},
|
|
postList: {
|
|
ZStack {
|
|
PostListView(selectedCollection: model.selectedCollection, showAllPosts: model.showAllPosts)
|
|
.withErrorHandling()
|
|
.frame(width: 300)
|
|
if model.isProcessingRequest {
|
|
ZStack {
|
|
Color(NSColor.controlBackgroundColor).opacity(0.75)
|
|
ProgressView()
|
|
}
|
|
}
|
|
}
|
|
},
|
|
postDetail: {
|
|
NoSelectedPostView(isConnected: $model.hasNetworkConnection)
|
|
}
|
|
)
|
|
.environmentObject(model)
|
|
.onChange(of: model.hasError) { value in
|
|
if value {
|
|
if let error = model.currentError {
|
|
self.errorHandling.handle(error: error)
|
|
} else {
|
|
self.errorHandling.handle(error: AppError.genericError())
|
|
}
|
|
model.hasError = false
|
|
}
|
|
}
|
|
#else
|
|
WFNavigation(
|
|
collectionList: {
|
|
CollectionListView()
|
|
.withErrorHandling()
|
|
},
|
|
postList: {
|
|
PostListView(selectedCollection: model.selectedCollection, showAllPosts: model.showAllPosts)
|
|
.withErrorHandling()
|
|
},
|
|
postDetail: {
|
|
NoSelectedPostView(isConnected: $model.hasNetworkConnection)
|
|
}
|
|
)
|
|
.environmentObject(model)
|
|
.onChange(of: model.hasError) { value in
|
|
if value {
|
|
if let error = model.currentError {
|
|
self.errorHandling.handle(error: error)
|
|
} else {
|
|
self.errorHandling.handle(error: AppError.genericError())
|
|
}
|
|
model.hasError = false
|
|
}
|
|
}
|
|
#endif
|
|
}
|
|
}
|
|
|
|
struct ContentView_Previews: PreviewProvider {
|
|
static var previews: some View {
|
|
let context = LocalStorageManager.standard.container.viewContext
|
|
let model = WriteFreelyModel()
|
|
|
|
return ContentView()
|
|
.environment(\.managedObjectContext, context)
|
|
.environmentObject(model)
|
|
}
|
|
}
|